有趣且实用的CSS小技巧
刷到一篇文章 有趣且实用的 CSS 小技巧 ,对一些 CSS 技巧感到有趣,于是打算自己试试,顺带记录一些相关 CSS 的 API,以便未来某些时刻用到。
打字效果
import React, { useState } from 'react'
import { css } from '@emotion/react'
export default function Typewriting() {
const TypewritingStyle = css`
.wrapper {
display: flex;
align-items: center;
justify-content: center;
}
.typing-demo {
width: 24ch;
animation: typing 2s steps(22), blink 0.5s step-end infinite alternate;
white-space: nowrap;
overflow: hidden;
border-right: 3px solid;
font-family: monospace;
font-size: 2em;
}
@keyframes typing {
from {
width: 0;
}
}
@keyframes blink {
50% {
border-color: transparent;
}
}
`
return (
<div css={TypewritingStyle}>
<div className='wrapper'>
<div className='typing-demo'>有趣且实用的 CSS 小技巧</div>
</div>
</div>
)
}
animation - CSS(层叠样式表) | MDN (mozilla.org)
主要使用到动画 animation 可分为两段
第一段: typing 2s steps(22) 在两秒内将文字分为22段显示,也就呈现打字的效果。
第二段:blink 0.5s step-end infinite alternate; 每0.5秒光标闪烁,无限交替
阴影效果
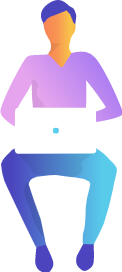
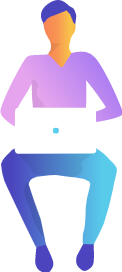
import { css } from '@emotion/react'
export default function Shadow() {
const ShadowStyle = css`
.wrapper {
display: flex;
align-items: center;
justify-content: center;
}
.mr-2 {
margin-right: 2em;
}
.mb-1 {
margin-bottom: 1em;
}
.text-center {
text-align: center;
}
.box-shadow {
box-shadow: 2px 4px 8px #585858;
}
.drop-shadow {
filter: drop-shadow(2px 4px 8px #585858);
}
`
return (
<div css={ShadowStyle}>
<div className='wrapper'>
<div className='mr-2'>
<div className='mb-1 text-center'>box-shadow</div>
<img className='box-shadow' src='https://markodenic.com/man_working.png' alt='Image with box-shadow' />
</div>
<div>
<div className='mb-1 text-center'>drop-shadow</div>
<img className='drop-shadow' src='https://markodenic.com/man_working.png' alt='Image with drop-shadow' />
</div>
</div>
</div>
)
}
平滑滚动
scroll-behavior - CSS(层叠样式表) | MDN (mozilla.org)
只需一行 CSS:scroll-behavior: smooth
截断文本
主要这三行代码
overflow: hidden;
white-space: nowrap;
text-overflow: ellipsis;
overflow: hidden; 溢出隐藏。
white-space: nowrap; 是强制显示为一行
text-overflow: ellipsis; 将文本溢出显示为(…)
如果元素没有宽度限制,那么不会省略,要多行省略可以使用 -webkit-line-clamp
需要浏览器提供支持
overflow: hidden;
text-overflow: ellipsis;
display: -webkit-box;
-webkit-line-clamp: 3;
-webkit-box-orient: vertical;
自定义滚动条
import { css } from '@emotion/react'
export default function ScrollBar() {
const ScrollBarStyle = css`
.wrapper {
display: flex;
align-items: center;
justify-content: center;
}
.tile {
overflow: auto;
display: inline-block;
background-color: #ccc;
height: 200px;
width: 200px;
}
.tile-content {
padding: 20px;
height: 500px;
}
.tile-scrollbar::-webkit-scrollbar {
width: 12px;
background-color: #eff1f5;
}
.tile-scrollbar::-webkit-scrollbar-track {
border-radius: 3px;
background-color: transparent;
}
.tile-scrollbar::-webkit-scrollbar-thumb {
border-radius: 5px;
background-color: #515769;
border: 2px solid #eff1f5;
}
`
return (
<div css={ScrollBarStyle}>
<div className='wrapper'>
<div className='tile tile-scrollbar'>
<div className='tile-content'>自定义滚动条</div>
</div>
</div>
</div>
)
}
::-webkit-scrollbar - CSS(层叠样式表) | MDN (mozilla.org)
有以下伪元素选择器去修改各式webkit浏览器的滚动条样式:
- ::-webkit-scrollbar — 整个滚动条.
- ::-webkit-scrollbar-button — 滚动条上的按钮 (上下箭头).
- ::-webkit-scrollbar-thumb — 滚动条上的滚动滑块.
- ::-webkit-scrollbar-track — 滚动条轨道.
- ::-webkit-scrollbar-track-piece — 滚动条没有滑块的轨道部分.
- ::-webkit-scrollbar-corner — 当同时有垂直滚动条和水平滚动条时交汇的部分.
- ::-webkit-resizer — 某些元素的corner部分的部分样式(例:textarea的可拖动按钮).
提示框
HTML/CSS tooltip
Hover Here to see the tooltip.
import { css } from '@emotion/react'
export default function Tooltip() {
const TooltipStyle = css`
.tooltip {
position: relative;
border-bottom: 1px dotted black;
}
.tooltip:before {
content: attr(data-tooltip);
position: absolute;
width: 100px;
background-color: #062b45;
color: #fff;
text-align: center;
padding: 10px;
line-height: 1.2;
border-radius: 6px;
z-index: 1;
opacity: 0;
transition: opacity 0.6s;
bottom: 125%;
left: 50%;
margin-left: -60px;
font-size: 0.75em;
visibility: hidden;
}
.tooltip:after {
content: '';
position: absolute;
bottom: 75%;
left: 50%;
margin-left: -5px;
border-width: 5px;
border-style: solid;
opacity: 0;
transition: opacity 0.6s;
border-color: #062b45 transparent transparent transparent;
visibility: hidden;
}
.tooltip:hover:before,
.tooltip:hover:after {
opacity: 1;
visibility: visible;
}
`
return (
<div css={TooltipStyle}>
<h1>HTML/CSS tooltip</h1>
<p>
Hover{' '}
<span className='tooltip' data-tooltip='Tooltip Content'>
Here
</span>{' '}
to see the tooltip.
</p>
</div>
)
}
使用到 CSS 函数 attr()
,可创建动态的纯 CSS 提示框
渐变边框(渐变色)
import { css } from '@emotion/react'
export default function Gradient() {
const GradientStyle = css`
.gradient-border {
border: solid 5px transparent;
border-radius: 10px;
background-image: linear-gradient(white, white), linear-gradient( 135deg, #81FFEF 10%, #F067B4 100%);;
background-origin: border-box;
background-clip: content-box, border-box;
}
.box {
margin: 0 auto;
width: 150px;
height: 100px;
display: flex;
align-items: center;
justify-content: center;
}
`
return (
<div css={GradientStyle}>
<div className='box gradient-border'>炫酷渐变边框</div>
</div>
)
}
使用 CSS 渐变 - CSS(层叠样式表) | MDN (mozilla.org)
列几个渐变色的网站
渐变色按钮: Buttons with CSS gradients - Gradient Buttons (colorion.co)
简单的配色网站: uiGradients - Beautiful colored gradients
提供大量配色: Gradient Colors Collection Palette - CoolHue 2.0 (webkul.github.io)